OAuth
- 作用 让”客户端”安全可控地获取”用户”的授权,与”服务商提供商”进行互动。
- 思路 OAuth在”客户端”与”服务提供商”之间,设置了一个授权层(authorization layer) 。”客户端”不能直接登录”服务提供商”,只能登录授权层,以此将用户与客户端区分开来。”客户端”登录授权层所用的令牌(token),与用户的密码不同。用户可以在登录的时候,指定授权层令牌的权限范围和有效期。”客户端”登录授权层以后,”服务提供商”根据令牌的权限范围和有效期,向”客户端”开放用户储存的资料。
OAuth 2.0 运行流程
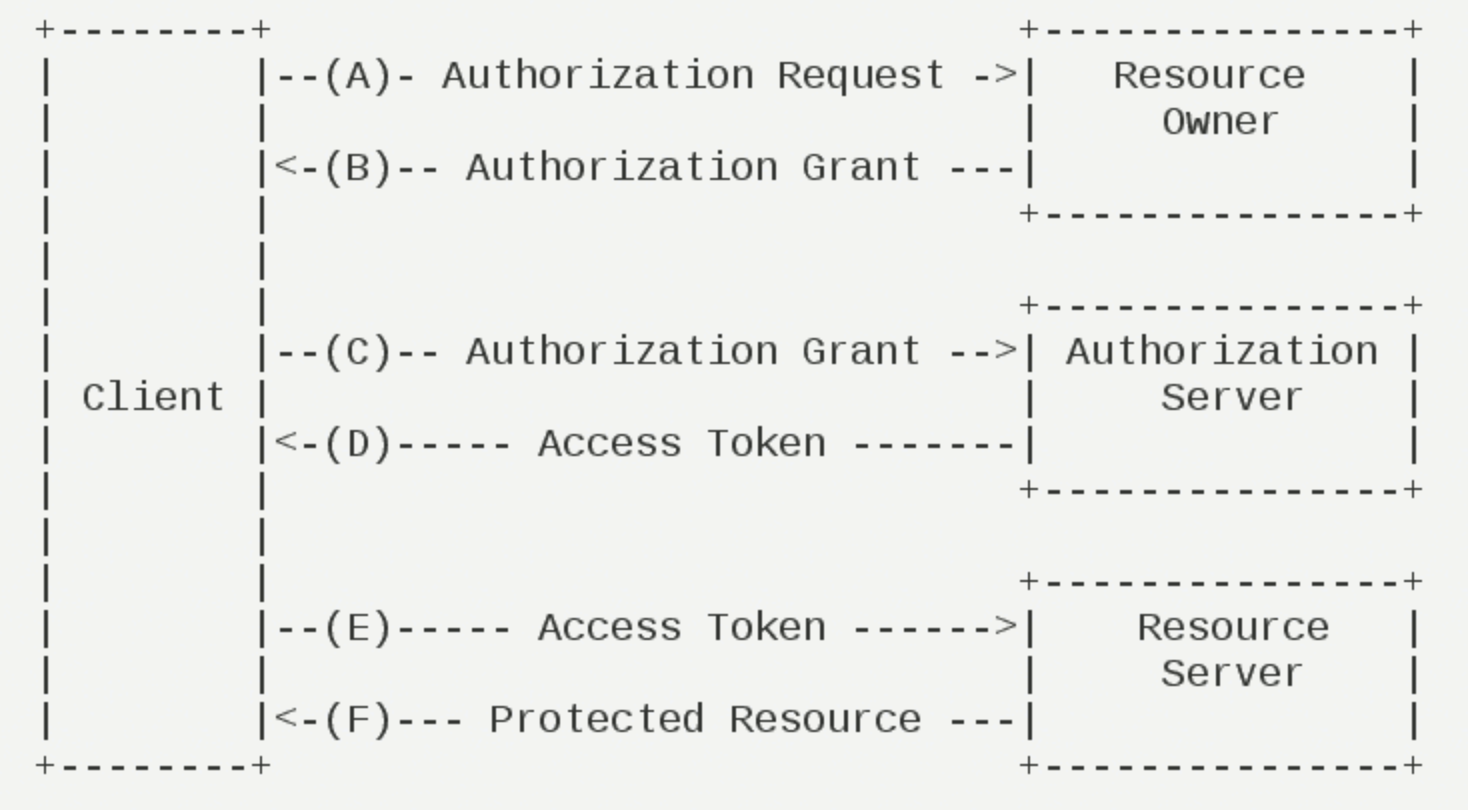
- A : 用户打开客户端以后,客户端要求用户给予授权。
- B : 用户同意给予客户端授权。
- C : 客户端使用上一步获得的授权,向认证服务器申请令牌。
- D : 认证服务器对客户端进行认证以后,确认无误,同意发放令牌。
- E : 客户端使用令牌,向资源服务器申请获取资源。
- F : 资源服务器确认令牌无误,同意向客户端开放资源。
OAuth 2.0 用户授权给客户端的方式
注意 不管哪一种授权方式,第三方应用申请令牌之前,都必须先到系统备案,说明自己的身份,拿到两个身份识别码:
客户端 ID(client ID)和客户端密钥(client secret)
- 授权码模式(authorization code)
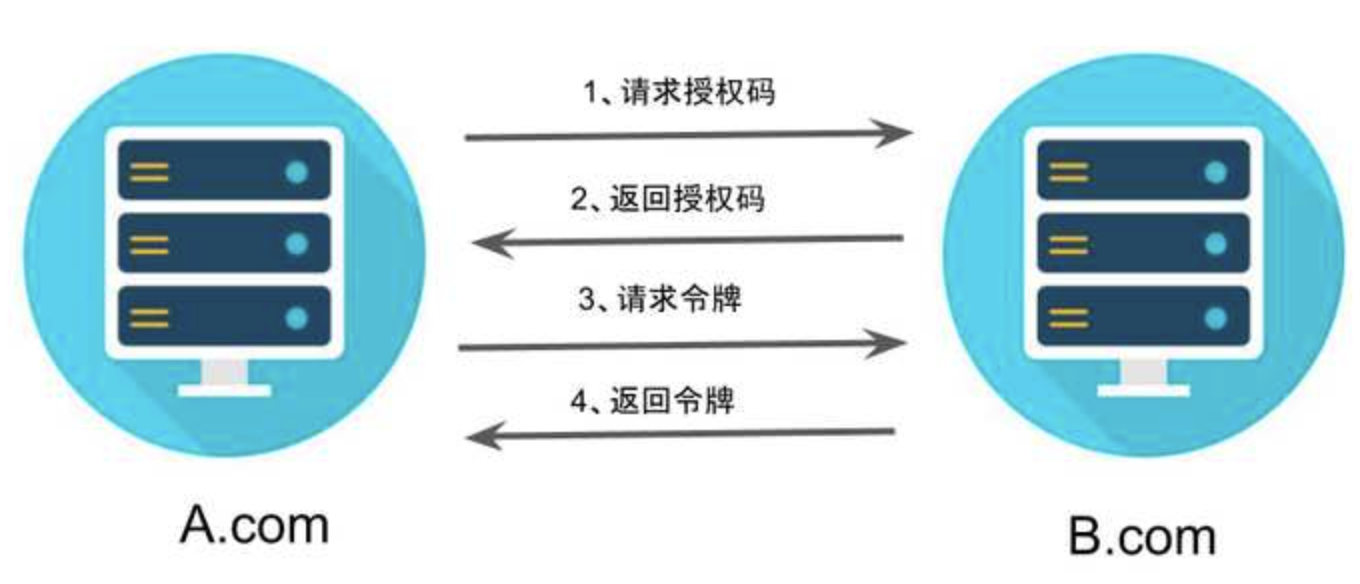
- 先申请一个授权码,再通过授权码获取令牌。适用于有后端的Web应用,最常用,安全性最高。授权码通过前端传送,令牌则是储存在后端,而且所有与资源服务器的通信都在后端完成。这样的前后端分离,可以避免令牌泄漏。
- 第一步 A 网站提供一个链接,用户点击后就会跳转到 B 网站,授权用户数据给 A 网站使用。示意链接:
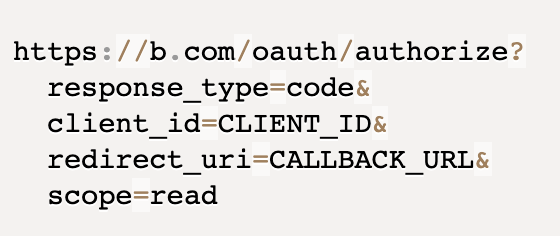
response_type=code 表示要求返回授权码,client_id 表示 是谁在请求, redirect_uri 表示B接收或拒绝A发起的请求后,跳转的地址 scope = read 表示要求的权限范围是只读。
第二步 用户点击链接跳转后,B网站会要求用户登录,并询问是否同意给予A的授权,同意后,跳转到redirect_uri指定的网址,跳转时,会携带一个授权码。 示意链接 :

- 第三步 A拿到授权码后,就可以在后端向B网站请求令牌。示意链接:
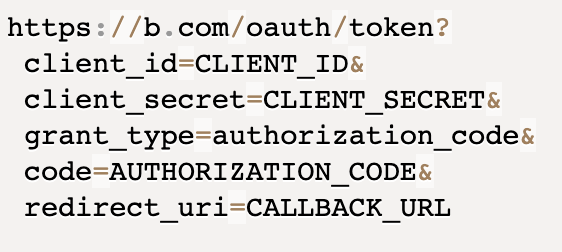
client_id 和 client_secret 用来让B确认A的身份,client_secret 是保密的,因此只能在后端发请求,grant_type=AUTHORIZATION_CODE 表示采用授权的方式是授权码方式,code 是之前拿到的授权码,redirect_uri 是令牌拿到后的回调网址
第四步 B 网站收到请求以后,就会颁发令牌。具体做法是向redirect_uri指定的网址,发送一段 JSON 数据。示意数据 :
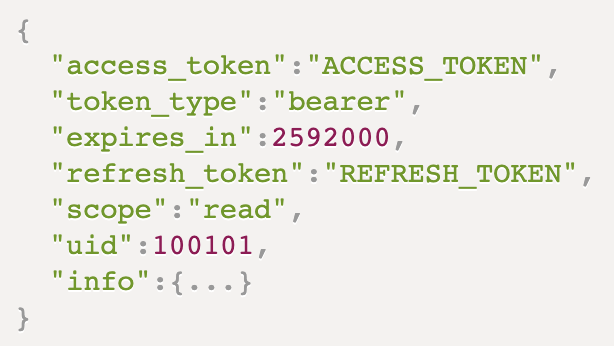
access_token 就是请求数据的令牌,expires_in 表示令牌过期时间 ,refresh_token就是刷新令牌的令牌
简化模式(implicit)
密码模式(resource owner password credentials)
客户端模式(client credentials)
使用令牌
- A 网站拿到令牌以后,就可以向 B 网站的 API 请求数据了。
- 此时,每个发到 API 的请求,都必须带有令牌。具体做法是在请求的头信息,加上一个Authorization字段,令牌就放在这个字段里面。
应用
github配置
- 生成Oauth应用
- 配置回调地址
公网访问本地应用
1 | ./ding -config=./ding.cfg -subdomain=cwww3 8080 |
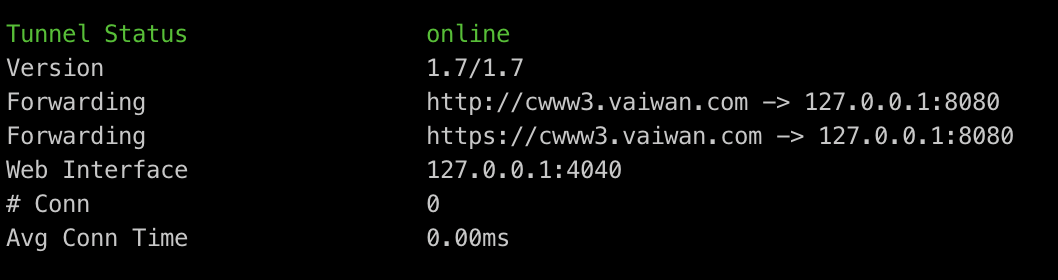
代码
- 本地程序
1 | package main |
效果
访问 http://cwww3.vaiwan.com/authorization
登录后 url 跳转
- 获得用户数据
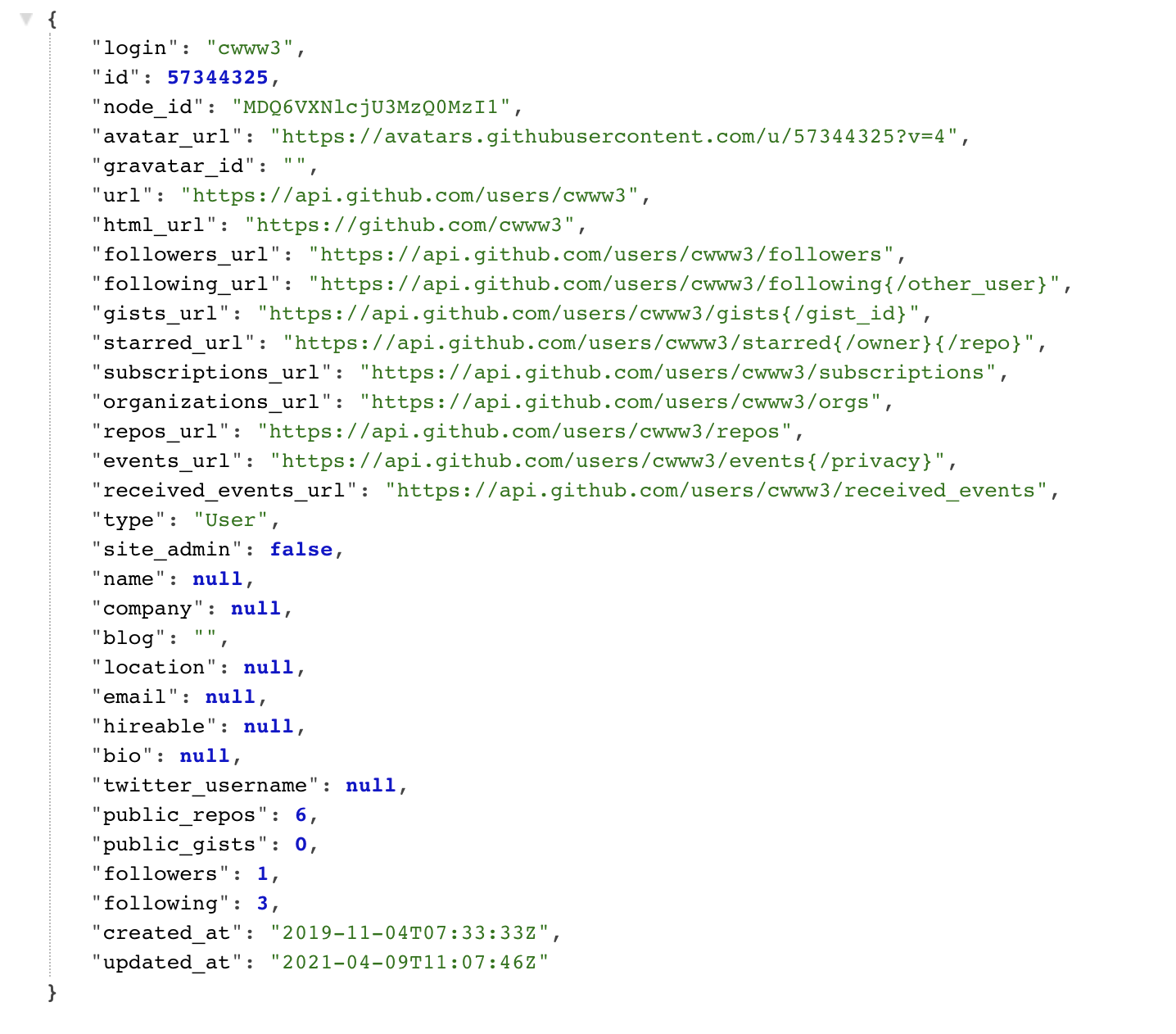